STRING METHODS
Method | Description |
capitalize() | Converts the first character to upper case |
casefold() | Converts string into lower case |
center() | Returns a centered string |
count() | Returns the number of times a specified value occurs in a string |
encode() | Returns an encoded version of the string |
endswith() | Returns true if the string ends with the specified value |
expandtabs() | Sets the tab size of the string |
find() | Searches the string for a specified value and returns the position of where it was found |
format() | Formats specified values in a string |
format_map() | Formats specified values in a string |
index() | Searches the string for a specified value and returns the position of where it was found |
isalnum() | Returns True if all characters in the string are alphanumeric |
isalpha() | Returns True if all characters in the string are in the alphabet |
isdecimal() | Returns True if all characters in the string are decimals |
isdigit() | Returns True if all characters in the string are digits |
isidentifier() | Returns True if the string is an identifier |
islower() | Returns True if all characters in the string are lower case |
isnumeric() | Returns True if all characters in the string are numeric |
isprintable() | Returns True if all characters in the string are printable |
isspace() | Returns True if all characters in the string are whitespaces |
istitle() | Returns True if the string follows the rules of a title |
isupper() | Returns True if all characters in the string are upper case |
join() | Joins the elements of an iterable to the end of the string |
ljust() | Returns a left justified version of the string |
lower() | Converts a string into lower case |
lstrip() | Returns a left trim version of the string |
maketrans() | Returns a translation table to be used in translations |
partition() | Returns a tuple where the string is parted into three parts |
replace() | Returns a string where a specified value is replaced with a specified value |
rfind() | Searches the string for a specified value and returns the last position of where it was found |
rindex() | Searches the string for a specified value and returns the last position of where it was found |
rjust() | Returns a right justified version of the string |
rpartition() | Returns a tuple where the string is parted into three parts |
rsplit() | Splits the string at the specified separator, and returns a list |
rstrip() | Returns a right trim version of the string |
split() | Splits the string at the specified separator, and returns a list |
splitlines() | Splits the string at line breaks and returns a list |
startswith() | Returns true if the string starts with the specified value |
strip() | Returns a trimmed version of the string |
swapcase() | Swaps cases, lower case becomes upper case and vice versa |
title() | Converts the first character of each word to upper case |
translate() | Returns a translated string |
upper() | Converts a string into upper case |
zfill() | Fills the string with a specified number of 0 values at the beginning |
TYPES OF STRINGS
1.SLICING STRING
String slicing allows you to extract a portion of a string by specifying the start and end indices. The start index is inclusive, meaning that the character at that index is included in the slice, while the end index is exclusive, meaning that the character at that index is not included in the slice.
Here’s an example of string slicing in Python:
message = "Hello, World!" substring = message[7:12] print(substring)
Output:
World
In this example, we have a string message
which contains the phrase “Hello, World!”. By using slicing, we extract a portion of the string starting from index 7 (inclusive) and ending at index 12 (exclusive). The resulting substring is assigned to the variable substring
and then printed, which outputs “World”.
You can also omit the start or end index to slice from the beginning or until the end of the string, respectively. Here are a few examples:
message = "Hello, World!"
substring1 = message[:5] # Slice from the beginning until index 5 (exclusive)
substring2 = message[7:] # Slice from index 7 (inclusive) until the end
substring3 = message[:] # Slice the entire string
print(substring1)
print(substring2)
print(substring3)
Output:
Hello
World!
Hello, World!
In the first example, we slice the string from the beginning until index 5 (exclusive), resulting in "Hello". In the second example, we slice the string from index 7 (inclusive) until the end, resulting in "World!". Lastly, we slice the entire string by omitting both the start and end indices, resulting in the original string "Hello, World!".
String slicing is a powerful tool for extracting substrings from strings and is commonly used in tasks such as parsing, text manipulation, and data extraction.
2.CONCATENATION OF STRING:
To concatenate, or combine, two strings you can use the + operator.For concatenation of string we use "+" symbol
Example 1:
Merge variable a with variable b into variable c:
a = "Hello"
b = "World"
c = a + b
print(c)
OUTPUT:
HelloWorld
Example 2:
To add a space between them, add a " ":
a = "Hello"
b = "World"
c = a + " " + b
print(c)
OUTPUT:
Hello World
June 2023
INTRODUCTION TO PYTHON STRINGS
Strings in Python are a fundamental data type used to represent and manipulate textual data. A string is a sequence of characters enclosed in single quotes (”), double quotes (“”) or triple quotes (”’ ”’).
Strings can be created by assigning a value enclosed in quotes to a variable.
For example:
message = "Hello, World!" Python treats strings as immutable objects, meaning that once a string is created, it cannot be modified. However, various string manipulation operations can be performed to extract information or create new strings. String concatenation is a common operation where two or more strings are combined to create a single string. This can be done using the + operator or by simply placing the strings next to each other. For example: greeting = "Hello" name = "Alice" message = greeting + ", " + name + "!" Python provides a rich set of built-in string methods for performing various operations on strings. These methods include 'upper()' and 'lower()' for changing the case of the string, 'split()' for splitting a string into a list of substrings, 'join()' for joining a list of strings into a single string, and many more. String formatting is used to create dynamic strings by incorporating variable values into the string. Python provides different approaches for string formatting, including the '%' operator, the 'format()' method, and f-strings. For example: name = "Bob" age = 25 message = "My name is %s and I'm %d years old." % (name, age) Python strings support a wide range of escape sequences, such as \n for a new line, '\t' for a tab, '\"' and '\' 'for including quotes within a string, and \\ for a literal backslash.June 2023
PYTHON KEYWORDS
In Python, keywords are reserved words that have special meanings and purposes in the language. These keywords cannot be used as identifiers (variable names, function names, etc.) because they have predefined meanings within the Python syntax. Here is a list of Python keywords:
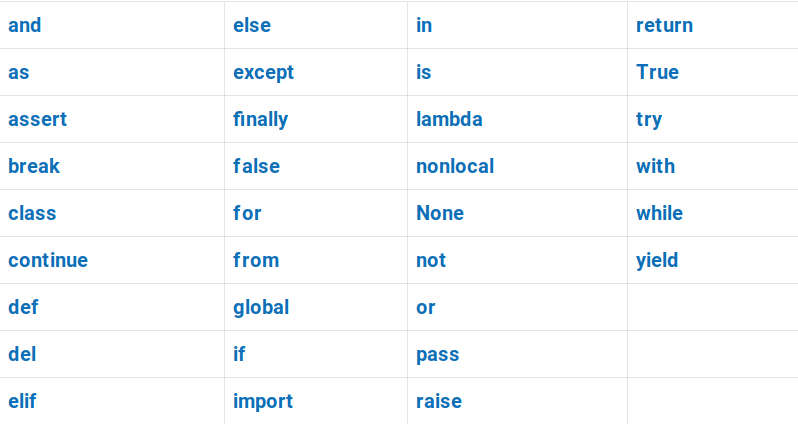
These keywords are an integral part of the Python language and are used to define the structure, flow, and behavior of Python programs. It's important to note that keywords are case-sensitive, so using variations in capitalization will not change their meaning. PROGRAM ON "FALSE": python # Checking if a number is positive number = -10 if number > 0: is_positive = True else: is_positive = False print(is_positive) OUTPUT: False EXPLANATION: In this example, we have a variable named 'number' which is assigned a value of -10. We want to check whether the number is positive or not. We use an 'if' statement to compare the 'number' with 0. If the number is greater than 0, we set the 'is_positive' variable to 'True'; otherwise, we set it to 'False'. Finally, we print the value of 'is_positive', which will be 'False' in this case, since -10 is not a positive number.
DISADVANTAGES OF PYTHON
- Slower Execution Speed: Python is an interpreted language, which means it may run slower compared to compiled languages like C or C++. The interpreter’s overhead and dynamic typing can result in slower execution speed for certain computationally intensive tasks. However, Python offers options for optimizing performance, such as using compiled extensions or integrating with other high-performance languages.
- Memory Consumption: Python’s dynamic typing and high-level abstractions come at the cost of increased memory consumption compared to lower-level languages. The overhead of objects and automatic memory management can result in higher memory usage, which may be a concern for memory-constrained systems or applications that handle large datasets. However, Python provides memory management tools like garbage collection, and memory optimization techniques can be applied to mitigate these concerns.
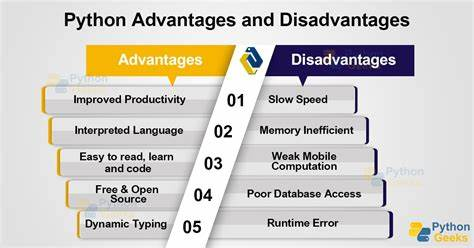
3.Version Compatibility: The transition from Python 2 to Python 3 introduced backward-incompatible changes, and Python 2.x is no longer officially supported since January 1, 2020. However, there are still legacy systems and libraries that rely on Python 2, which can create compatibility issues. Care must be taken when migrating from Python 2 to Python 3 or when integrating with older codebases.
4.Global Interpreter Lock (GIL): Python has a Global Interpreter Lock, commonly known as the GIL. The GIL allows only one thread to execute Python bytecode at a time, even on multi-core systems. This can limit the parallel execution of Python threads and potentially impact the performance of CPU-bound tasks. However, the GIL primarily affects CPU-bound code and doesn’t hinder the performance of I/O-bound or concurrent tasks.
5.Mobile and Browser Support: While Python is versatile and widely used, it is not as commonly supported for mobile app development as languages like Java or Swift. Additionally, running Python code directly in web browsers is not as straightforward as with languages like JavaScript. However, there are frameworks like Kivy and BeeWare that help in mobile app development, and tools like Brython and Transcrypt for running Python in the browser.
June 2023ADVANTAGES OF PYTHON
- Large and Active Community: Python has a vast and active community of developers worldwide. This community contributes to the development of libraries, frameworks, and tools, making Python a robust ecosystem. The availability of a wide range of open-source libraries and modules allows developers to leverage existing solutions, reducing development time and effort. The community also provides extensive documentation, tutorials, and support, making it easier to learn and troubleshoot Python.
- Extensive Libraries and Frameworks: Python has a rich collection of libraries and frameworks that simplify and accelerate development in different domains. Libraries like NumPy, Pandas, and Matplotlib provide powerful tools for scientific computing and data analysis. Web development frameworks like Django and Flask enable rapid and scalable web application development. Frameworks like TensorFlow and PyTorch are widely used in machine learning and artificial intelligence. Python’s extensive ecosystem allows developers to leverage existing solutions and focus on solving specific problems rather than reinventing the wheel.
- High-level language: Python abstracts away many low-level details, such as memory management and hardware operations, allowing programmers to focus on solving problems rather than worrying about intricate system-specific details. Python also provides a large standard library and numerous third-party libraries and frameworks, which further enhance its productivity and ease of use.
- Object–Oriented and Procedural Programming language.
- Portable and Interactive.
- Ideal for prototypes – provide more functionality with less coding.
- Highly Efficient (Python’s clean object-oriented design provides enhanced process control, and the language is equipped with excellent text processing and integration capabilities, as well as its own unit testing framework, which makes it more efficient.)
- Internet of Things(IoT) Opportunities
- Interpreted Language: In an interpreted language, the source code is executed line by line, with each line being interpreted and executed at runtime, without the need for prior compilation into machine code.The interpreted nature of Python makes it well-suited for tasks that require quick development, prototyping, and scripting. However, it’s worth noting that Python also provides options for optimizing performance, such as compiling Python code to bytecode or leveraging just-in-time (JIT) compilers for specific use cases.
EVOLUTION OF PYTHON
Birth (Early 1990s): Python was created by Guido van Rossum, a Dutch programmer, in the late 1980s, and its development continued into the early 1990s. It was first released to the public in 1991. This can be considered Python’s “birth” or infancy stage.
Early Childhood (1991-1994): During this phase, Python gained initial attention and started to gain popularity among early adopters. It was primarily used in academia and small-scale projects, slowly learning to crawl and take its first steps.
Adolescence (1994-2000): In this stage, Python started gaining wider recognition and acceptance within the programming community. Its simplicity, readability, and focus on code maintainability helped it stand out. The release of Python 2.0 in 2000 marked a significant milestone in its development, introducing many new features and improvements.
Adulthood (2000-2008): Python matured further during this period. Its community grew significantly, and the language continued to evolve with regular updates and enhancements. The introduction of Python 2.2 in 2001 brought garbage collection improvements and a new type hierarchy.
Full Maturity (2019-present): Python 3 eventually gained widespread adoption, and the community embraced the language’s modern version. Python’s popularity soared, driven by its versatility, extensive libraries, and its use in emerging technologies such as data science, machine learning, and web development. Python 3.8, released in 2019, introduced many new features, including assignment expressions and positional-only parameters.
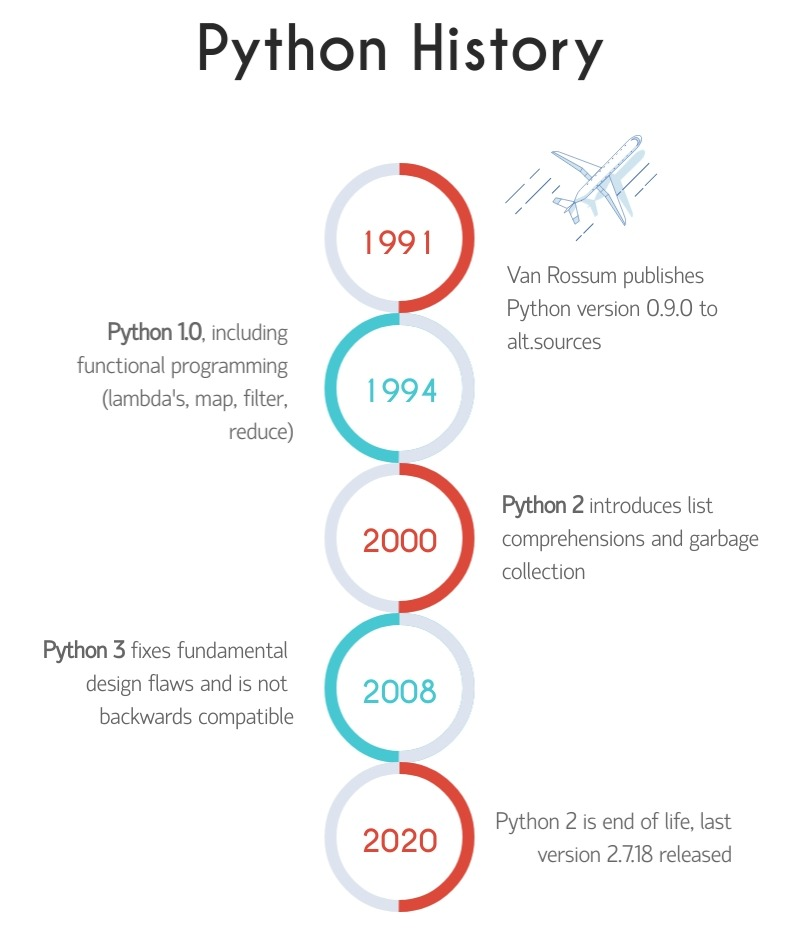
VERSIONS IN PYTHON:
- Python 1.x: The initial version of Python, with several subversions released between 1991 and 1994.
- Python 2.x: The next major release series, which had various subversions and updates. Notable versions include Python 2.0 (released in 2000), Python 2.4 (2004), Python 2.7 (2010). Python 2.7 marked the end of the Python 2.x series, with its end-of-life announced for January 1, 2020.
- Python 3.x: A significant and backward-incompatible release series, aimed at improving the language’s design and addressing various shortcomings. Notable versions include Python 3.0 (2008), Python 3.4 (2014), Python 3.6 (2016), Python 3.8 (2019). Python 3.9 (2020) and Python 3.10 (2021) were released after my knowledge cutoff.
- It’s important to note that Python 2.x and Python 3.x are not fully compatible, and transitioning from Python 2 to Python 3 required adapting code and addressing differences. The Python community encouraged the adoption of Python 3.x due to its improved features and support, and Python 2.x is now considered legacy.
- Each major version release introduced new features, syntax enhancements, performance improvements, and bug fixes, aiming to make Python more powerful and efficient. The community actively supports and maintains Python 3.x, with regular updates and improvements.
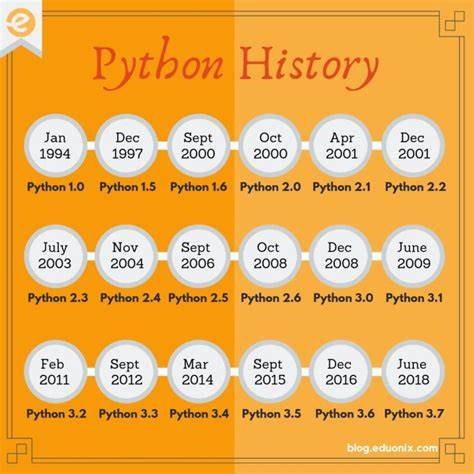
SILENT FEATURES OF PYTHON
☛ Code Quality
Python code is highly readable, which makes it more reusable and maintainable. It has broad support for advanced software engineering paradigms such as object-oriented (OO) and functional programming.
☛ Developer Productivity
Python has a clean and elegant coding style. It uses an english-like syntax and is dynamically-typed. So, you never declare a variable. A simple assignment binds a name to an object of any type. Python code is significantly smaller than the equivalent C++/Java code. It implies there is less to type, limited to debug, and fewer to maintain. Unlike compiled languages, Python programs don’t need compiling and linking, which further boosts the developer’s productivity.
☛Developer Productivity
Python has a clean and elegant coding style. It uses an english-like syntax and is dynamically-typed. So, you never declare a variable. A simple assignment binds a name to an object of any type. Python code is significantly smaller than the equivalent C++/Java code. It implies there is less to type, limited to debug, and fewer to maintain. Unlike compiled languages, Python programs don’t need compiling and linking, which further boosts the developer’s productivity.
☛ Code Portability
Since Python is an interpreted language, so the interpreter has to manage the task of portability. Also, Python’s interpreter is smart enough to execute your program on different platforms to produce the same output. So, you never need to change a line in your code.
☛ Built-In And External Libraries
Python packages a large no. of the prebuilt and portable set of libraries. You can load them as and when needed to use the desired functionality.
☛ Component Integration
Some applications require interaction across different components to support the end to end workflows. Onc such component could be a Python script while others be a program written in languages like Java/C++ or any other technology.
Python has several ways to support the cross-application communication. It allows mechanisms like loading of C and C++ libraries or vice-versa, integration with Java and DotNET components, communication using COM/Silverlight, and interfacing with USB devices over serial ports. It can even exchange data over networks using protocols like SOAP, XML-RPC, and CORBA.
☛ Free To Use, Modify And Redistribute
Python is an OSS. You are free to use it, make amends in the source code, and redistribute, even for commercial interests. It is because of such openness that Python has garnered a vast community base that is continually growing and adding value.
☛ Object-Oriented From The Core
Python primarily follows the object-oriented programming (OOP) design. OOP provides an intuitive way of structuring your code, and a solid understanding of the concepts behind it can let you make the most out of your coding. With OOP, it is easy to visualize the complex problem into smaller flows by defining objects and how they correlate. And then, we can form the actual logic to make the program work.
June 2023